How to Create Custom Functoid ?
Create the C# Class Library Project as mentioned below
Object : Creating Custom Functoid, which can add leading Zeros and right align for the input number.
Solution Name : ZeroFormater.sln
Project Name : ZeroFormater
Class : ZeroFormater.cs
ResourceFile : ZeroFormaterRes.resx
Before we start writing code, will try to understand the basic concepts of Custom Functoid.
I can understand reading the below content is too boring to you, however it would be better to recollect the concepts once.
I aimed to create a custom functoid which can accept any number and convert to Right align value with specified number of leading Zeros and decimal characters. So I called it as “ZeroFormater”.
If I want to convert 234.45 to 0000234.45 , I cannot use any of existing functoid provided by Microsoft unless I write scripting function(if you need this functionality for 2 or many times you do not have any choice to write same code ). I am trying to achieve this by creating custom functoid.
What is Custom Functoid ?
BizTalk provides a fairly full toolbox of functoids, which will sufficient for your needs. However you will encounter occasions where you can't find just the right operation for your needs. That is where custom functoids come in the picture.
You can create a custom functoid by deriving from the Microsoft.BizTalk.BaseFunctoid class (implemented by Microsoft.BizTalk.BaseFunctoids.dll )
Key things to be remember
Ø Functoid ID should start from 6000
Ø Use Resource to represent the Tooltip, Icon, Functoid Name and description.
Ø Use only 16x16 pixel bitmap Image for Functoid Icon
Ø Copy the DLL at \ Microsoft BizTalk Server 2006\Developer Tools\Mapper Extensions
Ø Attach Assembly key file to your project before you Build
Creating Class
Add a new class to your project and name it as ZeroFormater.cs and import the below namespaces
using Microsoft.BizTalk.BaseFunctoids;
using System.Reflection;
Create namespace as “ZeroFormater” and Public class as “RightalignZeroFormater”
Write the below code.
Add new Resource Item and rename it as ZeroFormaterRes.resx and add 3 new strings as mentioned below.
ZFORMATER_DESC : ZeroFormater accepts 3 parameters and it converts to Right align leading zero format.Fist parameter : Should be your input string. second parapter: Length of your sting before decimal place.Third parameter: How many characters after decimal place
ZFORMATER_NAME : ZeroFormater
ZFORMATER_TOOLTIP : RightAlignLeadingZerosFormater
Now Add new “BitMap File” to your project and rename it as ZFORMATER_BITMAP.bmp
Create a CustomFunctoid.snk and attach to your Solution. And write a single line of code to your
AssemblyInfo.cs as mentioned below.
[assembly: AssemblyKeyFile("CustomFunctoid.snk")]
Here the brief outlook of your solution after completing the above steps.
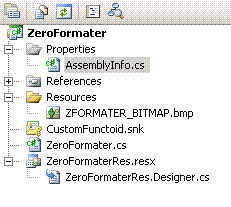
We are 50% of the work completed for creating custom functoid. Now lets start write the actual code.
namespace ZeroFormater
{
// Adding Zeros to your Input value and right aligned.
public class RightalignZeroFormater : BaseFunctoid
{
public RightalignZeroFormater()
{
this.ID = 6990;
this.SetupResourceAssembly("ZeroFormater.ZeroFormaterRes", Assembly.GetExecutingAssembly());
this.SetBitmap("ZFORMATER_BITMAP");
this.SetDescription("ZFORMATER_DESC");
this.SetName("ZFORMATER_NAME");
this.SetTooltip("ZFORMATER_TOOLTIP");
//Define the number of parameters to your Functoid
this.SetMinParams(3);
this.SetMaxParams(3);
//determines which function of the class will be used when the Functoid is invoked
this.SetExternalFunctionName(GetType().Assembly.FullName,
"ZeroFormater.RightalignZeroFormater", "GlobalDecimalFunctoid");
//defining category in toolbox, and decides under which category the
functoid will be added.
this.Category = FunctoidCategory.String ;
//define output
this.OutputConnectionType = ConnectionType.AllExceptRecord;
// Parameter 1
this.AddInputConnectionType(ConnectionType.AllExceptRecord);
// Paramter 2
this.AddInputConnectionType(ConnectionType.AllExceptRecord);
// Paramter 3
this.AddInputConnectionType(ConnectionType.AllExceptRecord);
}
Now we try to understand what this code is?
this.ID = 6990;
we I mentiond above the id should start from 6000, here I am using 6990 as my functoid id.
You need to set a number of input Parameters for your functoid.
this.SetMinParams(3);
this.SetMaxParams(3);
The below lines indicates that what is the Icon you would like to display at design time for your Functoid and what is your brief description about you functiod which en-user can understand(try to write short and user understablele words), Then Name of your functoid and tooltip.
this.SetBitmap("ZFORMATER_BITMAP");
this.SetDescription("ZFORMATER_DESC");
this.SetName("ZFORMATER_NAME");
this.SetTooltip("ZFORMATER_TOOLTIP");
Here you need to specify your actual function. Here I added my function name GlobalDecimalFunctoid which can add leading Zeros and right align format.
this.SetExternalFunctionName(GetType().Assembly.FullName,
"ZeroFormater.RightalignZeroFormater", "GlobalDecimalFunctoid");
this.Category = FunctoidCategory.String - which tab of the toolbox should display the functoid
Here you can see the functionality of GlobalDecimalFunctiod.
//Genarate Right Align Format.
public string GlobalDecimalFunctoid( string GValue,
Int16 LengthBeforeDecimal,
Int16 LengthAferDecimal
)
{
double dblValue = 0;
string GetTmpFormat = "";
if (GValue == "" GValue == null)
{
dblValue = 0;
}
else
{
dblValue = System.Convert.ToDouble(GValue);
}
GetTmpFormat = GenerateZeros(LengthBeforeDecimal) + "." + GenerateZeros(LengthAferDecimal);
return dblValue.ToString(GetTmpFormat);
}
//Generating Zeros
public string GenerateZeros(Int16 iCount)
{
string ConString = "";
for (Int16 i = 0; i < constring =" ConString" style="color:#3333ff;">return ConString;
}
Few more steps to complete the build. Lets write few lines of code to you ZeroFormaterRes.Designer.cs Class.
Add the below piece of lies at the end of the ZeroFormaterRes class which can helps to pick the Image from your resource File for your Custom functoid.
internal static System.Drawing.Bitmap ZFORMATER_BITMAP {
get {
object obj = ResourceManager.GetObject("ZFORMATER_BITMAP", resourceCulture);
return ((System.Drawing.Bitmap)(obj));
Also Select ZeroFormaterRes.resx file and Right Click and then select View Code. You might be seeing a XML file. Write the below lines which helps to pickup
" < name= " ZFORMATER_BITMAP " type="System.Resources.ResXFileRef, System.Windows.Forms">
Resources\ZFORMATER_BITMAP.bmp;System.Drawing.Bitmap, System.Drawing, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b03f5f7f11d50a3a
< / data> "
If you failed to mention your correct PublicKeyToken for your System.Drawing assembly, you may not find your colorful Image for your functoid. It looks as follows.
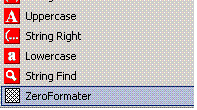
If you have a problem with Functoid image, you can do follow the below steps.
1. Restart the Windows Image Acquisition (WIA) Services
( click Start -> Run -> Services.msc)
2. Reset your BizTalk Tool Box
3. If you still face an issue – better you restart your system once.( biztalk always problem with memory refresh)
Final Steps
Build your Code and Copy your DLL to \ Microsoft BizTalk Server 2006\Developer Tools\Mapper Extensions
And GAC your assembly
Now Lets Try to use the Functoid at designer. Her is my Functoid Looks like.
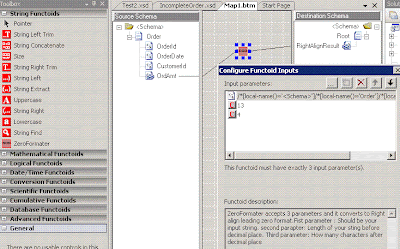
Functiond Inputs I entered 13 as my length of the string before Decimal point and 4 is the number after decimal point, below is my final Output.